10 Ways to Work with Large Files in Python: Effortlessly Handle Gigabytes of Data!
Handling large text files in Python can feel overwhelming. When files grow into gigabytes, attempting to load them into memory all at once can crash your program. But don’t worry — Python offers multiple strategies to efficiently process such files without exhausting memory or performance.
Whether you’re working with server logs, massive datasets, or large text files, this guide will walk you through the best practices and techniques for managing large files in Python. By the end, you’ll know how to handle gigabytes of data like a pro!
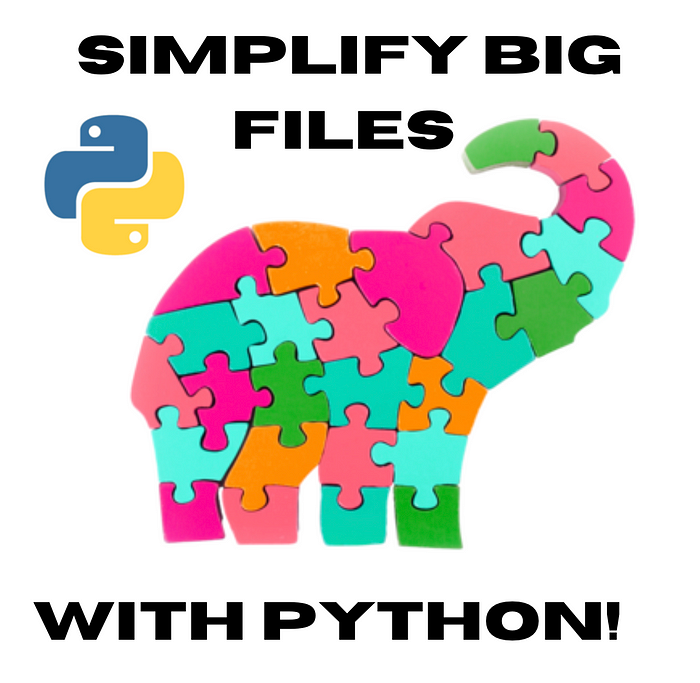
Why You Should Care About Working with Large Files
Large file processing isn’t just for data scientists or machine learning engineers. It’s a common task in many fields:
- Data Analysis: Server logs, transaction records, or sensor data often come in gigantic files.
- Web Scraping: Processing datasets scraped from the web.
- Machine Learning: Preparing training datasets that can’t fit into memory.
Key Benefits of Mastering These Techniques
- Avoid Memory Errors: Loading entire files into memory often leads to crashes (e.g.,
MemoryError
). - Faster Processing: By reading files incrementally, you can significantly boost performance.
- Resource Optimization: Run large-scale tasks even on machines with limited memory.\
10 Python Techniques to Handle Large Files
1. Using Iterators for Line-by-Line Reading
Reading a file line by line ensures only a small portion of the file is loaded into memory at any given time. Here’s how to do it:
with open('large_file.txt', 'r') as file:
for line in file:
process(line) # Replace with your processing function
- Why it works: Python treats the file object as an iterator, buffering small chunks of the file.
- Use case: Great for line-based logs, CSVs, or plain text.
2. Reading in Chunks
Sometimes, you need more flexibility than line-by-line reading. Reading a file in fixed-sized chunks gives you control over how much data you process at once.
def read_file_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
process(chunk) # Replace with your processing function
- Best for: Files where you don’t need line-by-line processing.
- Tip: Adjust
chunk_size
for optimal performance based on your system's memory.
3. Buffered File Reading
Buffered reading provides a higher level of optimization by processing files in larger internal chunks:
with open('large_file.txt', 'rb', buffering=10 * 1024 * 1024) as file: # 10 MB buffer
for line in file:
process(line)
Why use it? Reduces the overhead of frequent disk I/O operations.
4. Memory-Mapped Files (mmap)
Memory mapping allows Python to treat a file like a byte array directly in memory. It’s a game-changer for random access.
from mmap import mmap
with open('large_file.txt', 'r') as file:
with mmap(file.fileno(), length=0, access=mmap.ACCESS_READ) as mm:
for line in mm:
process(line.decode('utf-8'))
- When to use: For ultra-large files where you need random access.
- Bonus: Memory mapping can improve performance for read-heavy tasks.
5. Using Generators
Generators allow you to process data lazily, loading only what’s necessary.
def generate_lines(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
for line in generate_lines('large_file.txt'):
process(line)
Why it’s great: Reduces memory usage by processing one line at a time.
6. Processing Batches of Lines
For structured files, you can process groups of lines (or records) at once.
def read_batches(file_path, batch_size=5):
with open(file_path, 'r') as file:
batch = []
for line in file:
batch.append(line.strip())
if len(batch) == batch_size:
yield batch
batch = []
if batch:
yield batch
# Example usage:
for batch in read_batches('cars.txt'):
process_batch(batch) # Replace with your processing logic
Perfect for: Structured data like CSVs or logs.
7. Stream Processing
If data arrives continuously (e.g., logs or APIs), use stream processing.
import requests
def stream_data(url):
with requests.get(url, stream=True) as response:
for line in response.iter_lines():
process(line)
Use case: Real-time log monitoring or API data streams.
8. Dask for Parallel Processing
For massive datasets, consider Dask, a library designed for parallel computation on large data.
import dask.dataframe as dd
df = dd.read_csv('large_dataset.csv')
result = df[df['column'] > 100].compute()
Why Dask? Handles out-of-memory data by chunking it into smaller pieces.
9. PySpark for Distributed Processing
If your data size exceeds a single machine’s capacity, use PySpark for distributed processing.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("LargeFileProcessing").getOrCreate()
df = spark.read.csv('large_dataset.csv')
df.filter(df['column'] > 100).show()
Best for: Big Data tasks requiring cluster-level resources.
10. Efficient Libraries for Specific Formats
For specific file types, use optimized libraries:
- JSON:
ijson
for incremental JSON parsing. - XML:
lxml
for fast and memory-efficient XML parsing. - Parquet/Arrow:
pyarrow
orfastparquet
for columnar data.
Fun Facts About Large File Handling
- Memory-Efficient Python: Python uses lazy evaluation in many places (e.g., iterators) to minimize memory usage.
- Duck Typing: Python doesn’t care about the type of objects, just their behavior — a key reason why it excels in processing diverse data formats.
Common Mistakes to Avoid
- Loading the Entire File: Avoid
file.readlines()
unless the file is small. - Forgetting Buffering: Use buffered I/O for smoother performance.
- Ignoring Edge Cases: Always handle errors like empty lines or invalid formats.
Conclusion: Conquer Large Files in Python
Working with large files doesn’t have to be daunting. Whether you’re reading files line-by-line, processing chunks, or leveraging tools like Dask and PySpark, Python provides a rich set of tools for every need.
Which technique will you try first? Let me know in the comments below! And if you enjoyed this guide, don’t forget to follow me for more Python tips and tricks. Let’s tackle those gigabytes together! 🚀